In our increasingly digital world, the ability to convert image to text using Python has become an essential skill for developers, data scientists, and businesses alike. Image-to-text conversion, also known as Optical Character Recognition (OCR), is the process of extracting textual information from images, transforming visual data into machine-readable text. This technology bridges the gap between the physical and digital realms, enabling us to digitise vast archives of printed documents, extract information from screenshots and photos and much more. The applications of OCR are vast and continue to grow. From streamlining office workflows to powering cutting-edge artificial intelligence (AI) systems, the ability to perform image text extraction is transforming how we interact with visual information.
Python, with its rich ecosystem of libraries and tools, has emerged as a frontrunner in the OCR space. Its simplicity, coupled with powerful OCR engines like Tesseract and innovative libraries such as EasyOCR, makes Python an ideal choice for developers looking to implement OCR in their projects.
In this comprehensive guide, we'll dive deep into the world of Python OCR. We'll explore various techniques to convert image to text using Python, from basic implementations to advanced strategies for handling complex documents and multiple languages. Whether you're a beginner just starting with OCR or an experienced developer looking to enhance your text extraction capabilities, this guide will equip you with the knowledge and tools to master image-to-text conversion in Python.
Quick steps
- Install required libraries (pytesseract, Pillow, OpenCV)
- Import libraries in your Python script
- Load the image file
- Preprocess the image (optional but recommended)
- Use pytesseract.image_to_string() to extract text
- Print or process the extracted text
Introduction to image-to-text conversion
Image-to-text conversion, also known as Optical Character Recognition (OCR), is the process of extracting textual content from images. This technology bridges the gap between visual and digital information, allowing computers to "read" and process text found in photographs, scanned documents, and other image formats. At its core, OCR works by analysing the patterns of light and dark in an image to identify individual characters. The process typically involves six phases, from image preprocessing in Python OCR to character segmentation, feature extraction, character classification and post-processing to refine the output. While the concept of OCR dates back to the early 20th century, it has seen significant advancements with the rise of digital computing and, more recently, machine learning and AI, making the evolution of OCR technology one that continues to reshape how we interact with visual information.
Why use Python for OCR?
Python has become the go-to language for OCR implementations, and for good reason. Here’s why it's an excellent choice to convert image to text Python:
- Robust OCR libraries: Powerful libraries like Tesseract, easyOCR, and OpenCV text recognition offer advanced text extraction capabilities.
- Ease of use: Python's clean syntax makes complex OCR tasks accessible to both beginners and experts.
- Image processing tools: Libraries such as OpenCV enable crucial image preprocessing to enhance OCR accuracy.
- Machine learning integration: Seamlessly incorporate AI techniques to improve text recognition, especially for handling complex images in Python OCR.
- Cross-platform compatibility: Python OCR solutions work consistently across different operating systems.
- Active community: Extensive resources and support available for various OCR libraries and techniques.
- Scalability: Suitable for projects ranging from small scripts to large-scale enterprise applications.
- Continuous improvement: Regular updates to OCR libraries keep Python at the forefront of text extraction technology.
Popular Python libraries for image-to-text conversion
Tesseract OCR and OpenCV
Tesseract is a widely used open-source OCR (Optical Character Recognition) engine that provides accurate text extraction from images. You can use this library to convert image to text using Tesseract. Open Source Computer Vision Library (OpenCV) is a machine learning software library that provides various functionalities and algorithms to work with images and videos. OpenCV is written in C++ and offers interfaces for various programming languages, including Python.
You can use Tesseract and OpenCV to extract information from images using Python.
Setup
To begin, install Tesseract on your system. You can install it by following the instructions specific to your operating system.
Once Tesseract is set up, you must install the pytesseract library, which acts as a Python wrapper for Tesseract along with OpenCV.
pip install pytesseract
pip install opencv-python
After installing everything, follow the following steps for converting the text image to string using Tesseract.
Code Example
easyOCR
easyOCR is a user-friendly and efficient Python library for OCR. It provides a simple interface to extract text from images Python that are basic. To get started with easyOCR for text extraction, you need to install the library by running the following command:
Once installed, follow this simple easyOCR python tutorial to extract text from an image.
PyOCR
PyOCR is a Python wrapper that provides access to various OCR engines such as Tesseract, CuneiForm, and GOCR. It offers a unified interface to utilise these engines for text extraction from images. Here's an example of how to use PyOCR with Tesseract.
OCRopus
OCRopus is a collection of OCR tools and libraries developed by Google. It provides a framework for OCR research and includes various components like layout analysis, character recognition, and post-processing. OCRopus can be used for both single-page and multi-page document OCR. Here's a basic example.
These Python libraries offer additional options and flexibility regarding OCR in Python. Depending on your specific requirements and the nature of your images, exploring these alternative libraries might provide you with different features and performance characteristics.
Limitations of python libraries
Open source python libraries give good results for basic images but often fail for complex images. For example, they give inaccurate results if:
- The background is pixellated, blurry or same colour as the text.
- Image is a scanned copy of handwritten text.
- Image has multiple columns or irregular text placement.
They also cannot perform natural language processing (NLP) to check and improve the output. For example, if only partial text is extracted, NLP can guess and complete the results for better output. But python libraries cannot do this. They return incorrect results if the input is not standard.
Step-by-step guide: converting image to text
Step 1: Setting up your environment
Before you begin, ensure you have Python installed on your system. Then, set up your environment:
- Open a terminal or command prompt
- Install the necessary libraries:
pip install pytesseract pillow opencv-python
- Download and install Tesseract OCR engine from the official Github repository
- Add Tesseract to your system PATH
Step 2: Preprocessing images for better results
Image preprocessing is crucial for improving OCR accuracy. Here are some common techniques:
Grayscale conversion:
import cv2
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
Noise reduction:
denoised_image = cv2.fastNlMeansDenoising(gray_image)
Thresholding:
_, binary_image = cv2.threshold(denoised_image, 0, 255, cv2.THRESH_BINARY + cv2.THRESH_OTSU)
Deskewing:
coords = np.column_stack(np.where(binary_image > 0))
angle = cv2.minAreaRect(coords)[-1]
if angle < -45:
angle = -(90 + angle)
else:
angle = -angle
(h, w) = binary_image.shape[:2]
center = (w // 2, h // 2)
M = cv2.getRotationMatrix2D(center, angle, 1.0)
rotated = cv2.warpAffine(binary_image, M, (w, h), flags=cv2.INTER_CUBIC, borderMode=cv2.BORDER_REPLICATE)
Sep 3: Implementing OCR with python
Now, let’s implement the OCR process:
import pytesseract
from PIL import Image
import cv2
import numpy as np
# Load the image
image_path = 'path/to/your/image.jpg'
image = cv2.imread(image_path)
# Preprocess the image
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
denoised_image = cv2.fastNlMeansDenoising(gray_image)
_, binary_image = cv2.threshold(denoised_image, 0, 255, cv2.THRESH_BINARY + cv2.THRESH_OTSU)
# Perform OCR
text = pytesseract.image_to_string(binary_image)
# Print the extracted text
print(text)
Use cases for image-to-text conversion
Image-to-text conversion has a wide range of applications across various industries. As organisations increasingly deal with vast amounts of visual data, the need for efficient Python OCR solutions has grown exponentially. Let’s explore some key use cases:
1. Document digitisation and data entry automation
Many organisations possess large volumes of scanned operational paperwork. These image scans contain valuable information that is not readily searchable, editable or analysable. By using Python text extraction techniques, businesses can convert this image data into string format, making it usable for storage, analysis and processing. For example, companies can extract supplier information, invoice dates and amounts from invoice images using OCR Python libraries. This extracted data can be stored for tax purposes, audits or to analyse supplier performance, significantly reducing manual data entry efforts.
2. Healthcare record management
The healthcare industry benefits greatly from optical character recognition Python technology. Python image processing for OCR enables the digital conversion of medical records, scans, and images. This process enhances accessibility, streamlines record-keeping, and facilitates quicker patient data retrieval, ultimately improving healthcare delivery. For example, handwritten notes are common in the healthcare industry. From writing down patients’ personal information, diagnoses and symptoms to prescriptions and follow-up recommendations. By using Python text extraction, you can easily digitise these handwritten notes to enhance the accuracy and accessibility of patient information, which reduces the risk of misplacing important medical notes.
3. Human resources (HR) and recruitment
HR departments can leverage Python OCR libraries to digitise resumes, application forms, and other recruitment-related documents. This allows for easier candidate information management, automated sorting, and quicker applicant screening processes. For example, when a new employee is onboarded, there are countless documents that are required, including but not limited to an employment contract, tax forms and company policies. By using image-to-text conversion using OCR, this streamlines the onboarding process by reducing manual data entry and ensures all necessary documentation is accurately captured and stored in the HRIS (Human Resource Information System). It allows for quicker preparation of onboarding packages and reduces the risk of missing critical information.
4. ID verification and authentication
OCR plays a crucial role in automating the verification of identification documents. Using Python OCR accuracy improvement techniques, systems can extract and verify information from passports, voter IDs, and rental agreements as part of authentication workflows, enhancing security measures and reducing manual verification time. For example, businesses with customer onboarding and KYC processes gather a number of identification documents, including passports, driver’s licenses and national ID cards. Using text-to-image conversion can speed up this process, automatically scanning, extracting and verifying critical information, accelerating customer onboarding.
5. Product information management
In the retail and food industry, OCR can be used to scan food labels and extract ingredient information when adding new products to databases. This process ensures accurate product listings and helps maintain up-to-date nutritional information. For example, during inventory management, item details such as stock levels, barcode numbers, storage locations and supplier information need to be recorded. This can be easily achieved by image-to-text extraction, enhancing inventory tracking and accuracy and reducing stockouts and overstock situations. Most python OCR solutions also integrate seamlessly with inventory management systems to automate reorder processes.
Handling different image formats and languages
When it comes to image-to-text conversion using Python, versatility is key. Modern OCR libraries support a wide range of image formats and languages, making them powerful tools for diverse text extraction tasks.
Supported image formats
Technically, you can extract text from all types of images in Python. Python OCR libraries like Tesseract and easyOCR can handle various image formats, including PNG, JPEG, TIFF, and BMP. However, the code complexity and output accuracy can vary greatly depending on the input you expect. You may just need a few lines of code if you expect an input of simple images, like the ones shown below. Such images have large text, less words, simple font and clear contrast between text and images.
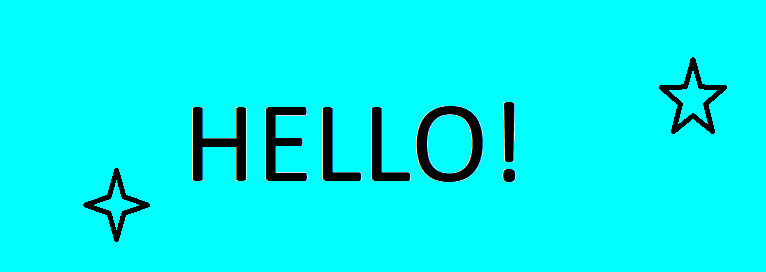
However, most text extraction input images have noisy backgrounds, varying fonts, shading or skewing of image text or handwritten text, like the one shown below.

Such images are going to require much more coding and testing efforts in a DIY coding program. You have to preprocess the text before extraction and then further analyze and correct the text after extraction.
For example, when using OpenCV’s text recognition, you can easily convert between formats:
import cv2
# Read image
image = cv2.imread('input.png')
# Convert to JPEG
cv2.imwrite('output.jpg', image)
Not all images are going to be in English, either. So, it’s important to use a solution that supports multiple languages for accurate text-to-image extraction.
Supported languages
Python OCR libraries offer extensive language support, making them versatile tools for global text extraction needs. Here's an overview of language support in popular OCR libraries:
- Tesseract OCR: Supports over 100 languages, including major world languages and many lesser-known ones. It can handle both left-to-right and right-to-left scripts.
- easyOCR: As of 2024, easyOCR supports more than 80 languages, including complex scripts like Chinese, Japanese, Korean, and Arabic.
- Google Cloud Vision API: While not a standalone Python library, it can be accessed via Python and supports over 50 languages for OCR.
To use different languages with Tesseract, you need to install the appropriate language data files. For example, to perform OCR on German text:
import pytesseract
from PIL import Image
pytesseract.pytesseract.tesseract_cmd = r'path_to_tesseract_executable'
text = pytesseract.image_to_string(Image.open('german_text.png'), lang='deu')
With easyOCR, you can specify multiple languages when initialising the reader:
import easyocr
reader = easyocr.Reader(['en', 'ch_sim', 'ja']) # English, Simplified Chinese, Japanese
result = reader.readtext('multilingual_image.png')
Working with multiple languages
When working with multi-language OCR in Python, it's important to consider the specific requirements of each language, such as character set, reading direction, and any special preprocessing needs. Multi-language OCR Python capabilities have significantly improved, allowing for efficient text extraction from diverse linguistic sources. Here's a simple example of multi-language OCR using easyOCR:
import easyocr
reader = easyocr.Reader(['en', 'fr']) # Initialize for English and French
result = reader.readtext('multilingual_image.png')
Dealing with non-Latin scripts
Python OCR libraries have made great strides in handling non-Latin scripts, such as Chinese, Arabic, or Cyrillic. When working with these scripts, it's essential to use the appropriate language models and sometimes apply specific preprocessing techniques. For example, with Tesseract:
import pytesseract
from PIL import Image
pytesseract.pytesseract.tesseract_cmd = r'path_to_tesseract_executable'
text = pytesseract.image_to_string(Image.open('arabic_text.png'), lang='ara')
Improving OCR accuracy for complex documents
Extracting text from complex documents can be challenging, but several techniques can significantly improve OCR accuracy.
Image pre-processing techniques
Pre-processing is crucial for enhancing OCR accuracy. Python image processing for OCR often involves techniques such as:
- Binarization
- Noise reduction
- Deskewing
- Contrast adjustment
Post-processing and error correction
After extracting text, post-processing can correct common OCR errors:
- Spell-checking
- Context-based correction
- Regular expression patterns for expected formats (e.g., dates, phone numbers)
Combining multiple OCR engines
To further improve OCR accuracy, especially when handling complex images in Python OCR, consider combining results from multiple engines. This approach can leverage the strengths of different OCR libraries:
import pytesseract
import easyocr
def combine_ocr_results(image_path):
# Tesseract OCR
tesseract_text = pytesseract.image_to_string(image_path)
# EasyOCR
reader = easyocr.Reader(['en'])
easyocr_result = reader.readtext(image_path)
easyocr_text = ' '.join([text for _, text, _ in easyocr_result])
# Combine results (implement your own logic here)
combined_text = combine_texts(tesseract_text, easyocr_text)
return combined_text
Alternative image-to-text conversion methods: cloud APIs and third party AI tools
While Python libraries offer powerful OCR capabilities, they're not the only option for converting images to text. In recent years, cloud-based APIs and specialised third-party AI tools have emerged as strong alternatives, offering state-of-the-art accuracy and scalability. These solutions can be particularly appealing for businesses and developers looking for high-performance OCR technology without the need for extensive local setup or maintenance.
Cloud APIs
You can use fully managed OCR services provided by cloud providers for extracting text from images. The cloud providers handle the underlying complexity of text extraction. You pass the image to the API as input and get the string as output. The top three cloud OCR services are:
You can call any API in your code based on the cloud infrastructure of your organization. Below we give an example of Google Cloud Vision API. First, set up a Google Cloud Project.
- Visit the Google Cloud Console and create a new project.
- Enable the Cloud Vision API for the project.
- Generate an API key or set up authentication credentials to access the API.
After setting up, follow the code example steps below.
The API analyses the image and returns the extracted text and additional information, such as bounding box coordinates and confidence scores.
Third party AI tools
Third party AI tools provide more comprehensive, AI powered solutions for image processing. For example, Affinda’s document AI platform powers many custom image extraction solutions like invoice processing, recruitment data extraction and ID data extraction. It combines three AI techniques:
- Computer vision technologies
- Deep learning
- Natural language processing
All three work together to pre-process documents, extract text and post-process results. All you need to do is input the image and then you can use the output as you like. Affinda offers off-the-shelf solutions, like their NextGen Resume Parser or Invoice Extractor, or their team of AI experts can develop a custom solution to suit your specific business needs. Price and usage are totally under your control!
Latest advancements in Python OCR (2024)
The field of Python OCR is experiencing rapid evolution, with several groundbreaking developments enhancing its capabilities and applications:
New features in Tesseract 5.3.0
Tesseract has released version 5.3.0 with several improvements:
- Enhanced neural network models: The latest version incorporates advanced deep learning models, significantly improving accuracy for both printed and Python OCR for handwritten text recognition.
- Improved layout analysis: Tesseract now offers better handling of complex document structures, including multi-column layouts and tables.
- Expanded language support: The engine now supports over 100 languages, with improved accuracy for non-Latin scripts.
- Performance optimisation: Faster processing speeds and reduced memory usage make it more efficient for large-scale OCR tasks.
Improvements in easyOCR's language support
easyOCR, known for its user-friendly API, has made significant strides in multilingual support:
- Expanded language coverage: As of 2024, easyOCR supports over 80 languages, including complex scripts like Chinese, Japanese, and Arabic.
- Enhanced accuracy for non-Latin scripts: Improved models for languages with intricate character sets have significantly boosted recognition accuracy.
- Multi-language detection: easyOCR can now automatically detect and process text in multiple languages within the same image.
Integration of AI models for enhanced accuracy
The integration of advanced AI and machine learning models has transformed OCR accuracy:
- Large Language Models (LLMs): OCR systems now leverage LLMs for context understanding and error correction, significantly improving accuracy in challenging scenarios.
- Computer Vision AI: Integration with state-of-the-art computer vision models has enhanced image preprocessing and character recognition.
- Adaptive Learning: Some OCR systems now incorporate adaptive learning algorithms that improve over time based on user corrections and feedback.
Real-time OCR capabilities
Advancements in processing power and algorithms have enabled real-time OCR applications:
- Mobile OCR: Optimised libraries like OpenCV and TensorFlow Lite allow for instant text recognition on mobile devices.
- Video stream processing: Real-time OCR can now be applied to video streams, opening up new possibilities in augmented reality and live translation services.
- Edge computing integration: OCR processing at the edge reduces latency and enables offline functionality for real-time applications.
OCR-as-a-service solutions
Cloud-based OCR services have become more sophisticated and accessible:
- Scalable Cloud APIs: Major cloud providers offer Python-compatible OCR APIs that can handle large volumes of documents with high accuracy.
- Specialised Industry Solutions: Tailored OCR services have emerged for specific sectors like healthcare (for medical records) and finance (for processing financial documents).
- Customisable Models: Some services allow users to fine-tune OCR models for specific document types or industry jargon, improving accuracy for niche applications.
Conclusion: automating business workflows with image-to-text conversion
As we've explored throughout this comprehensive guide, converting image to text using Python has become an increasingly powerful and accessible technology. From the basics of OCR to handling complex documents and multiple languages, Python offers a robust ecosystem of libraries and tools for text extraction tasks.
The latest advancements in 2024 have pushed the boundaries of what's possible with OCR, introducing AI-powered models, real-time processing capabilities, and improved accuracy for challenging scenarios like handwriting recognition. These developments have expanded the applicability of OCR across various industries, from digitising historical documents to automating data entry in modern businesses.
As OCR technology continues to evolve, it's crucial for developers and businesses to stay informed about the latest tools and techniques. Whether you're using established libraries like Tesseract or exploring cutting-edge solutions that integrate with large language models (LLMs), the key to success lies in choosing the right approach for your specific needs.
Remember that while OCR has come a long way, it's not without its challenges. Proper image preprocessing, post-processing error correction, and sometimes combining multiple OCR engines can significantly improve results.
As you consider integrating this technology into your business processes, consider the specific requirements of your use case, the languages you need to support, and the complexity of your documents. If you're looking for a tailored solution for your business, get in touch with our team today to see how we can help.
The future of using technology to convert image to text is bright, with ongoing research and development promising even more accurate and versatile text extraction capabilities. By leveraging the power of Python and staying abreast of the latest advancements, you'll be well-equipped to tackle any image-to-text conversion challenge that comes your way.